ReactJS Tutorial!
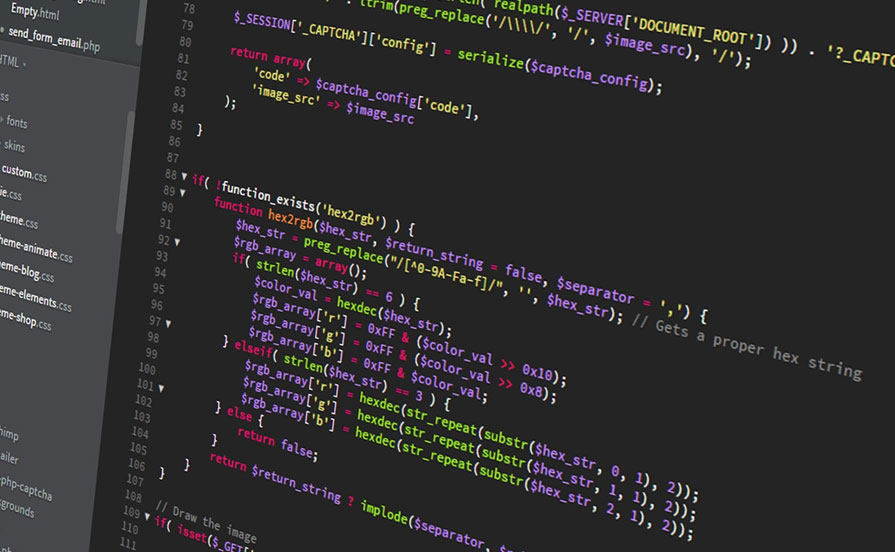
React is one of the most popular web frameworks out there. It has been growing steadily in popularity. This blog will show you how to create your own React website in just a few minutes.
Prerequisites:
To complete these steps you'll need to have the Node Package Manager (npm) installed. If you don't have it installed yet, head on over to https://nodejs.org/en/download/ to download and install npm.
Then open your command prompt and run npm install -g npx
We will use npx to run create-react-app
.
Create React App:
Create React App is an excellent way to quickly get a React website up and running. Create React App was created by Meta aka Facebook (the same company that created React!). In their docs, they describe it as: Create React App is an officially supported way to create single-page React applications. It offers a modern build setup with no configuration. Knowing that Create React App is supported by the creators of React is a huge plus. Let's use it to get started with our website!
Run the following command to create your site: npx create-react-app hello-react
(Note that it may take a couple minutes for this command to complete.)
Viewing the React website:
Next, run the following commands to start the React development server:
npm start
At this point a browser tab should open showing your React site. If it doesn't, visit http://localhost:3000 in your favorite browser to see your React site!
Updating the site:
Now, let's make a change to update the site. Open the hello-react/src/App.js
file, then replace the following line:
Edit <code>src/App.js</code> and save to reload.
with
My first React website!
If you open the web page again you'll see that it updated without you having to refresh the page! Live reloading is one of the awesome features that Create React App configures for you.
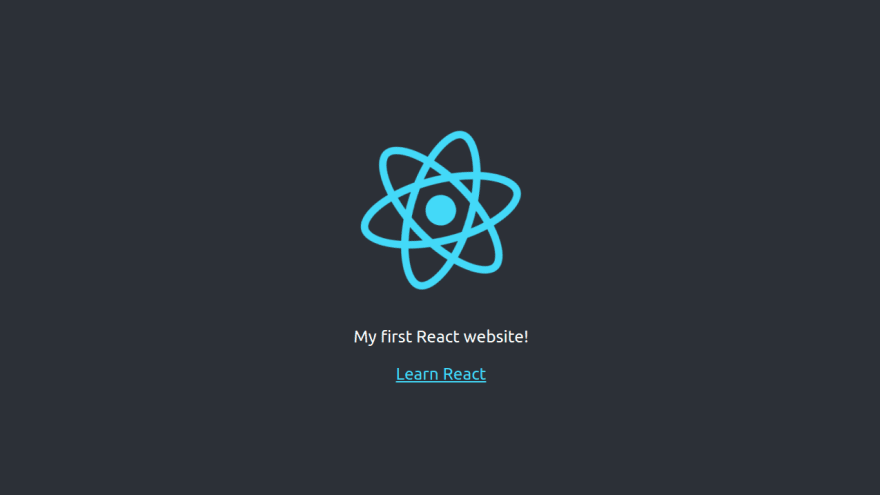
Creating a React Component:
Next, we'll create a new React component. First, create a folder in the src
folder named components
. Then create a file called HomepageImage.js
in the src/components
folder. This file will hold our new homepage image component.
We'll create this component by adding the following code to the HomepageImage.js
file:
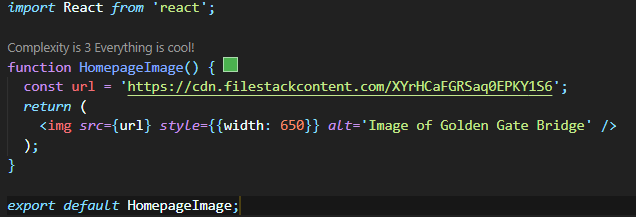
Then, in App.js
, replace

with

We also need to import the component at the top of App.js
by adding the following code to the top of the file:

Since we removed the image of the React logo, you can then remove this import for the logo as well:
import logo from './logo.svg';
The final App.js
file should look like this:
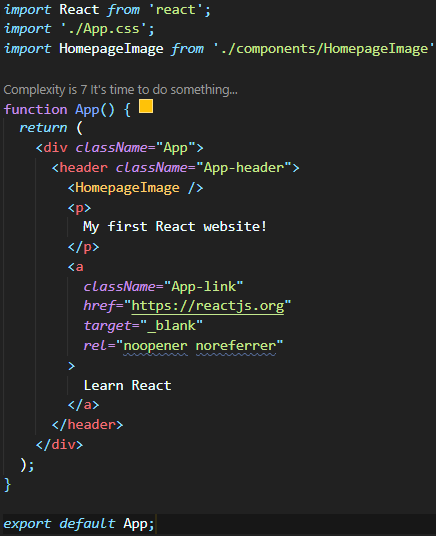
Now, open http://localhost:3000 again in your browser. If everything is working, you should see the following page:
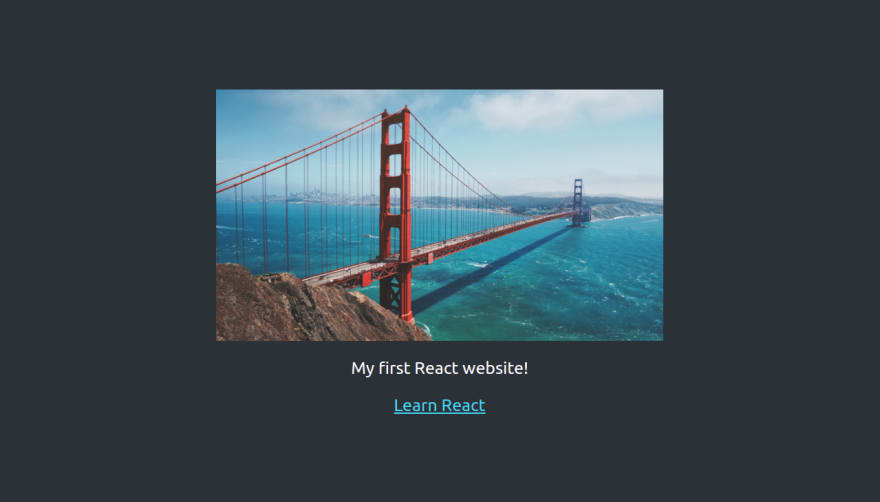
Next Steps:
This blog post was a quick introduction to creating web pages with React. If you want to gain a better understanding of React so you can build awesome sites using it, checkout Official ReactJS Documentation for better learning.
EXTRA:
What is React?
React is an open-source, reusable component-based, front-end JavaScript library used for building interactive UIs.
It was developed at Facebook as knows as Meta in 2011.
In an MVC architecture, React is the View, which is responsible for how the application looks and feels.
React uses declarative approach of programming which makes the code more predictable and easier to debug.
Now, let’s understand the meanings of declarative approach and imperative approach of programming.
In imperative approach, the application designer clearly specifies how the work needs to be accomplished or how the application needs to be executed.
Whereas, in declarative approach, the application designer simply specifies what needs to be accomplished and leaves it to the framework to decide how the work is going to be accomplished.
Note — We have to remember that React is not a framework, rather its a library which uses declarative approach of programming.
Features of React:
- JSX — JavaScript Syntax Extension — JSX is a syntax extension to JavaScript. In React, it is used to describe how the UI should look like. With the help of JSX, we can write HTML code in the same file that contains JavaScript code.
- Virtual DOM — React creates a virtual DOM (or VDOM) which is the exact copy of the real DOM, but it is lightweight. Manipulating real DOM is much slower than manipulating the VDOM. For example — when the state of an object changes, i.e., when we render a JSX element, every single VDOM object gets updated. This process is very quick as compared to updating all the objects in the real DOM. Once the VDOM gets updated, React compares the updated VDOM with the VDOM snapshot that was taken right before the update. By comparing them, React figures out exactly which VDOM object has been changed. Finally, React updates only those objects in the Real DOM.
Thank you for taking the time to read my blog... I hope it helped you along your journey in coding in react!